How Do Microcontrollers Run Python?
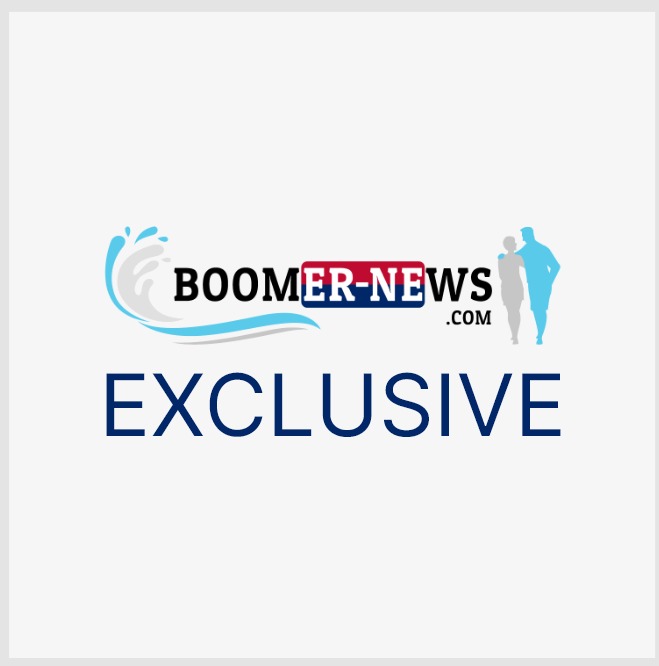
Imagine a tiny device with the power to execute high-level Python code in real time—transforming simple instructions into complex hardware actions. This isn’t science fiction; it’s the reality of microcontrollers running Python. In this article, we’ll peel back the layers to reveal how Python is made to work on constrained hardware, compare MicroPython with traditional Python, and show you practical steps to get the most out of your projects.
info: "The magic lies in the interpreter—a streamlined translator that takes your Python code and converts it into machine-level instructions in real time."
1. The Journey from High-Level Code to Machine-Level Execution
1.1 Understanding the Interpreter
When you write Python on your computer, you’re usually relying on a full-featured interpreter. Microcontrollers, however, have far fewer resources, so they need a version of Python that’s lean and efficient—enter MicroPython (or its sibling, CircuitPython).
How it Works:
- Writing Code: You create a script using familiar Python syntax.
- Uploading the Script: Your code is transferred to the microcontroller.
- The MicroPython Interpreter: This tiny interpreter parses your code, converting it into a series of low-level, hardware-friendly instructions.
- Execution: The microcontroller executes these instructions directly, despite having limited memory and processing power.
Here’s a simple code snippet to blink an LED:
import machine
import time
led = machine.Pin(2, machine.Pin.OUT)
while True:
led.value(1) # Turn LED on
time.sleep(0.5)
led.value(0) # Turn LED off
time.sleep(0.5)
This concise code hides a sophisticated process: every line is optimized to run in a fraction of the memory available on the microcontroller.
info: "MicroPython bridges the gap between high-level programming and low-level hardware control, enabling rapid prototyping without sacrificing performance."
2. Traditional Python vs. MicroPython: What Sets Them Apart?
2.1 Memory Footprint and Performance
Traditional Python runs on machines with gigabytes of RAM and fast processors. In contrast, many microcontrollers work with as little as 64KB of RAM and a fraction of the clock speed. To make Python work here, MicroPython eliminates non-essential libraries and features, ensuring that every byte counts.
-
Memory Stats:
- Typical Microcontroller: 64KB RAM, 256KB Flash
- Desktop Computer: 4GB+ RAM, multi-gigahertz processors
These constraints require MicroPython to be extremely efficient in both memory management and code execution.
2.2 Library Support and Use Cases
Standard Python comes loaded with extensive libraries for data analysis, web development, and more. MicroPython, however, is built primarily for interacting with hardware—sensors, motors, and LEDs. For instance, if you’re interested in integrating AI into your hardware projects, learning the fundamentals is a great start. Check out AI Engineering for deeper insights into leveraging AI in constrained environments.
Similarly, when you need your microcontroller to interact with external systems, our API Programming course provides practical strategies for bridging the gap between hardware and online services.
3. Under the Hood: Translating Python to Machine Code
3.1 The Conversion Process
The process of turning Python code into something the microcontroller can understand involves several steps:
- Parsing and Tokenizing: The interpreter reads your code, breaking it down into tokens.
- Bytecode Generation: These tokens are converted into bytecode—a low-level set of instructions.
- Execution: The microcontroller’s CPU then executes this bytecode directly, ensuring every instruction is optimized for the limited hardware.
This method is remarkably similar to how traditional Python works, but it’s heavily streamlined. To see it in action, let’s look at another code example that reads sensor data:
import machine
import time
adc = machine.ADC(machine.Pin(34))
adc.atten(machine.ADC.ATTN_11DB) # Configure input attenuation for full range
while True:
sensor_value = adc.read() # Read sensor value
print("Sensor reading:", sensor_value)
time.sleep(1)
info: "Every command you write is a carefully orchestrated instruction—translated from Python into a language the hardware can execute, all while operating within severe memory and processing constraints."
3.2 Stats and Performance Figures
Consider these benchmarks:
- Execution Speed: Microcontrollers typically operate in the range of tens of megahertz.
- Memory Efficiency: Optimized code in MicroPython can run using less than 50% of available memory, leaving room for crucial real-time operations.
These stats underscore why every line of code matters in such a constrained environment.
4. Navigating the Constraints: Memory and Resource Management
4.1 Handling Limited RAM
Memory management is key when working with microcontrollers. With such limited RAM, MicroPython employs several strategies:
- Lean Interpreter: Only core functionalities are included.
- Efficient Data Structures: Variables and objects are stored in the smallest possible footprint.
- Garbage Collection: Unused memory is automatically freed to avoid overload.
4.2 Practical Coding Tips
Here are some actionable strategies for writing efficient code:
- Optimize Your Code: Keep functions short and focused.
- Minimize Library Usage: Only import what you need.
- Monitor Memory Usage: Use debugging tools to track memory consumption.
For those diving deeper into related fields, integrating database interactions can be a game changer. Explore practical guides on databases in our Database resource. This understanding not only applies to hardware projects but also enhances your overall programming skills.
Furthermore, if you’re curious about advanced integration techniques between hardware and external services, our API Programming content provides step-by-step examples and best practices.
5. Practical Resources and Next Steps
5.1 Expand Your Knowledge Base
As you embark on projects with microcontrollers, consider supplementing your learning with these resources:
- For Advanced AI Applications: The AI Engineering course offers insights into integrating machine learning models on constrained hardware.
- APIs and External Services: Strengthen your backend integration skills with the API Programming course.
- Data Handling: For robust data management techniques, visit our Database guide.
5.2 Hands-On Practice
Experiment with code examples and start small. Begin with a blinking LED, then move on to reading sensors and integrating external APIs. Real-world practice will solidify your understanding and reveal how every microsecond and byte of memory is managed.
info: "Every experiment you run is a stepping stone towards mastering the art of constrained computing—where every line of code matters and every byte counts."
5.3 Community and Continuous Learning
Join forums, subscribe to newsletters, and participate in coding challenges. Engaging with a community can provide you with invaluable insights and support. And remember, every project is an opportunity to learn something new about the hidden logic that powers your microcontroller.
For more in-depth technical guidance on AI applications, revisit our AI Engineering resource. If you’re focused on integrating microcontrollers with web services, our API Programming tutorials are a must-read. Also, don’t miss our comprehensive Database guide for managing data effectively.
6. Conclusion: Empower Your Projects with In-Depth Knowledge
Microcontrollers are small yet powerful—capable of executing complex Python code with astonishing efficiency. By understanding how the MicroPython interpreter translates high-level code into machine-level instructions, you gain the power to optimize every project you undertake.
Keep these key takeaways in mind:
- Efficiency is Key: Optimize your code to work within strict memory limits.
- Practical Knowledge Matters: Hands-on experience, combined with the right resources, is the best way to learn.
- Continuous Learning: Leverage community resources and advanced courses like AI Engineering, API Programming, and Database to stay ahead of the curve.
Embrace the journey of blending high-level programming with low-level hardware control. Every project is an opportunity to innovate, learn, and push the boundaries of what a microcontroller can do. Now is the time to take action—experiment, iterate, and build something truly extraordinary!
More Tutorials
Explore these eBook tutorials to learn the basics of electronics:
The Ultimate Guide to Electronics: A full guide to learning electronics basics.
Op-Amps Made Simple: Learn how op-amps work in a simple way.
How Transistors Secretly Power Your Entire Life!: Understand what transistors do in everyday electronics.
Semiconductors: The Tiny Material That Runs the World!: Get a clear look at semiconductors and their role.
Resistors Explained!: See why resistors are needed in circuits.
Inductor – The Key to Controlling Energy in Circuits!: Find out how inductors work.
Diodes: The One Tiny Component That Changed Electronics Forever!: Learn about diodes and their everyday use.
Digital Electronics Made Simple: Understand digital electronics in easy steps.
Capacitor – Why They’re in Every Electronic Device!: Learn why capacitors are important.
Analogue Electronics – Learn How It Really Works!: A simple guide to analogue electronics.
The Ultimate Circuit Design Guide: Learn how to design circuits step by step.
Check Here More Tutorials: Have an nice experience learning all.
- On different context, for side-hustle, you can check this too. It'll be worth your time and effort.
- Also it comes with pre-made blueprint of steps so that you don't have to dig things from bottom
Money with AI & Print-on-Demand
???? Passive Income with AI & Print-on-Demand – The Ultimate Bundle!Turn AI-generated designs into profit-making print-on-demand products with this step-by-step bundle! Whether you're just starting out or looking to scale, this all-in-one package gives you everything you need to launch and automate your AI-powered POD business.What’s Included???? The Ultimate Guide 20+ page in-depth ebook covering: How to use AI tools (Midjourney, Canva, Kittl) to create designs Step-by-step process for creating and selling print-on-demand products like T-shirts, mugs, posters, and more Detailed strategies for product research, automation, and scaling your business ???? Bonus Checklist Printable, step-by-step checklist to keep you organized: Niche selection and market research Design creation and store setup Marketing, automation, and scaling strategies Everything you need to stay on track ???? Exclusive ChatGPT Prompts 22+ optimized prompts for every stage of your journey: Generate AI designs and optimize them for print Create compelling product listings, ad copy, and social media posts Automate your workflow and streamline operations Why This Bundle is a Game-Changer Save Time & Effort: Follow a proven strategy without endless research. No Design Skills Needed: Leverage AI to create stunning designs. Low Overhead, High Potential: Build a passive income stream with minimal upfront costs. Multi-Platform Compatibility: Perfect for use with Etsy, Shopify, Redbubble, Printful, Printify, and more. Get your copy now and start making money today!
Also YOU GET 50% OFFER ONLY PURCHASING HERE: Link