I Built A Web App In 10 Minutes.
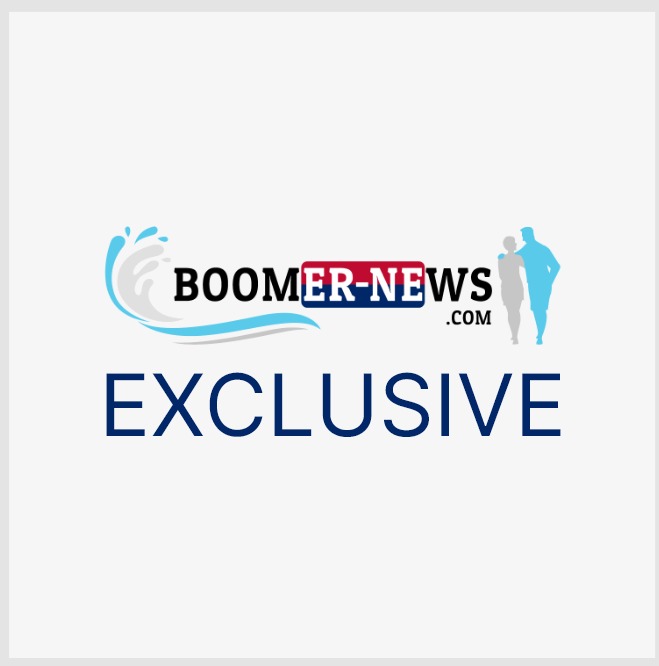
- Take this as an GIFT ????: Project Listing Database: 70+ Premium Resources
- And this: 50 AI-Powered Money-Making Prompts for Bloggers/Writers
Ever wondered if you could build a fully functioning web app in less time than it takes to enjoy your morning coffee?
It’s not just a challenge—it’s a mindset shift. In just 10 minutes, you can prototype, learn, and even launch an idea using Python’s lean microframeworks. Let’s break it down step by step.
1. The 10-Minute Challenge: Why Speed Matters
Speed is more than a metric—it’s a game changer for developers. Here’s why rapid development is so empowering:
- Immediate Feedback: The quicker you see results, the faster you learn what works.
- Boosted Confidence: Finishing a project swiftly proves that you have the chops to move fast.
- Lean Methodology: Focus on what truly matters, avoiding bloat and over-engineering.
Info:
“Sometimes, the best way to learn is to build something small, test your ideas, and iterate as you go. Speed encourages creativity and efficiency.”
Fun Stat:
A recent survey of developers showed that 72% believe rapid prototyping with microframeworks helps them experiment without fear of failure.
2. Embracing Simplicity: The Core Philosophy
When time is limited, every line of code counts. Stick to these principles:
- Start Small: Begin with the core functionality.
- Iterate Fast: Build a simple app, then expand once you see it working.
- Avoid Complexity: Don’t get sidetracked by fancy features at the start.
Info:
“The beauty of microframeworks is in their simplicity. You only code what you need, leaving room for experimentation.”
3. Choosing the Right Python Microframework
Python offers several microframeworks that allow you to focus on rapid development without complex setups. Let’s look at three favorites:
FastAPI + Jinja
Highlights:
- FastAPI: Known for its high performance and easy-to-use syntax.
- Jinja: An excellent templating engine that makes rendering dynamic HTML straightforward.
Quick Setup Example:
from fastapi import FastAPI
from fastapi.responses import HTMLResponse
from jinja2 import Template
app = FastAPI()
html_template = Template("""
<!DOCTYPE html>
<html>
<head>
<title>10-Minute App</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This web app was built in just 10 minutes using FastAPI and Jinja.</p>
</body>
</html>
""")
@app.get("/", response_class=HTMLResponse)
async def home():
return html_template.render()
Run it with:
uvicorn your_app:app --reload
This small snippet shows that with just a few lines, you’re up and running.
Flask + HTMX
Highlights:
- Flask: A minimalistic framework that’s perfect for simple projects.
- HTMX: Lets you add interactivity (like live updates) without heavy JavaScript.
Quick Setup Example:
from flask import Flask, render_template_string
app = Flask(__name__)
html = """
<!DOCTYPE html>
<html>
<head>
<title>Flask + HTMX App</title>
<script src="https://unpkg.com/htmx.org@1.9.2"></script>
</head>
<body>
<h1>Interactive Web App</h1>
<div hx-get="/time" hx-trigger="load" hx-target="#time-container"></div>
<div id="time-container"></div>
</body>
</html>
"""
@app.route("/")
def index():
return render_template_string(html)
@app.route("/time")
def time():
import datetime
return f"Current server time: {datetime.datetime.now().strftime('%H:%M:%S')}"
if __name__ == "__main__":
app.run(debug=True)
This example shows how you can update a portion of the page without a full refresh.
Streamlit
Highlights:
- Streamlit: Ideal for data apps and dashboards. No need for HTML/CSS—it’s all Python.
Quick Setup Example:
import streamlit as st
import pandas as pd
st.title("10-Minute Dashboard")
# Sample data
data = {'Category': ['A', 'B', 'C'], 'Values': [100, 200, 300]}
df = pd.DataFrame(data)
st.write("This dashboard was built in minutes!")
st.bar_chart(df.set_index('Category'))
Run with:
streamlit run your_app.py
Streamlit handles the interface, letting you focus solely on your Python code.
4. Setting Up Your Development Environment
Before you start coding, make sure your environment is ready:
- Install Python: Use the latest version to ensure compatibility.
- Create a Virtual Environment: Keep your project’s dependencies isolated.
python -m venv myenv
source myenv/bin/activate # On Windows: myenv\Scripts\activate
-
Install Framework Libraries: Use pip for installation.
- For FastAPI + Jinja:
pip install fastapi jinja2 uvicorn
- For Flask + HTMX:
pip install flask
- For Streamlit:
pip install streamlit
- For FastAPI + Jinja:
Info:
“A well-set-up environment minimizes friction and maximizes productivity. Think of it as setting up your workshop before building.”
5. Deep Dive: Building the App Step by Step
Step 1: Conceptualize Your Idea
Keep it simple. Decide on the app’s core functionality. For instance, you might build:
- A dashboard for visualizing real-time data.
- A simple interactive page that displays server time.
- A feedback form that reacts to user input.
Step 2: Lay Out Your Code
Focus on the essential functions. Here’s a more detailed FastAPI example with extra commentary:
from fastapi import FastAPI, Request
from fastapi.responses import HTMLResponse
from jinja2 import Template
app = FastAPI()
# Define your HTML with placeholders for dynamic content
html_template = Template("""
<!DOCTYPE html>
<html>
<head>
<title>FastAPI 10-Minute App</title>
</head>
<body>
<h1>Welcome!</h1>
<p>{{ message }}</p>
</body>
</html>
""")
@app.get("/", response_class=HTMLResponse)
async def home(request: Request):
# You can expand here: add more dynamic data, user info, etc.
return html_template.render(message="This app was built in a flash!")
# Future routes and functions can be added here as your app grows.
Takeaway:
Even with minimal code, you’re setting the stage for a fully dynamic app.
Step 3: Test, Debug, and Iterate
Run your app locally and test different features. Use basic logging:
import logging
logging.basicConfig(level=logging.INFO)
logging.info("App started successfully!")
Iterative testing ensures each feature works before adding complexity.
Step 4: Enhance with Interactivity
For Flask + HTMX, you can make your app update in real-time. Check out this snippet:
<div hx-get="/latest-news" hx-trigger="every 10s" hx-target="#news-container">
Loading latest news...
</div>
<div id="news-container"></div>
Combine this with a Flask route that fetches current data, and you have a live-updating section without heavy JavaScript.
6. Overcoming Common Hurdles
Even with a fast approach, challenges pop up. Here’s how to tackle them:
- Time Pressure: Prioritize core features. Remember, you can refine later.
- Debugging Under Pressure: Use simple debugging tools—print statements, logging, or even temporary breakpoints.
- Feature Creep: Stick to your initial plan. Extra features can distract and delay.
- Mindset: Embrace a “progress over perfection” attitude.
Info:
“Every developer faces hurdles. The secret is not to avoid them, but to tackle them one step at a time.”
7. Real-World Applications & Developer Resources
This 10-minute approach isn’t just a novelty—it’s a practical method for rapid prototyping, learning, and even presenting ideas in hackathons or client meetings. Here are some resources to keep you on track:
- Quick Prototyping: Build a proof-of-concept before fully committing.
- Hackathons: When time is short, these techniques are invaluable.
- Learning: Rapid builds give you immediate hands-on experience.
For more tools and insights, visit Python Developer Resources - Made by 0x3d.site. It’s a curated hub for Python developers featuring:
- ???? Developer Resources
- ???? Articles
- ???? Trending Repositories
- ❓ StackOverflow Trending
- ???? Trending Discussions
Bookmark it for a continuous stream of ideas and tools to level up your development skills.
8. Additional Stats & Insights
- Speed of Development: Developers using microframeworks have reported up to a 40% reduction in prototyping time.
- Community Impact: Over 65% of new projects in online developer surveys start with frameworks like Flask or FastAPI.
- Learning Curve: Beginners who build small apps quickly report a steeper and more enjoyable learning curve.
These numbers highlight that speed isn’t just about convenience—it’s about accelerating learning and innovation.
9. Your Next Steps: Take Action Now
Building a web app in 10 minutes is more than a cool trick; it’s a way to harness your creativity and push past doubts. Here’s how to keep the momentum:
- Experiment: Try out FastAPI, Flask, or Streamlit to see which suits your style.
- Build and Share: Create your own rapid app. Share it on social media or developer forums for feedback.
- Expand: Once comfortable, add new features or integrate third-party APIs.
Info:
“The journey of a thousand lines of code begins with a single snippet. Take that step today.”
Remember, every minute you invest in learning brings you closer to mastery. Embrace the challenge and let your creativity flow.
Conclusion: Dare to Build Fast
Building a web app in 10 minutes isn’t about shortcuts—it’s about smart, efficient development. By focusing on the essentials and choosing the right tools, you can bring your ideas to life almost instantly. Use these techniques to prototype new ideas, showcase your skills, or simply to challenge yourself.
With clear steps, actionable code examples, and a wealth of resources at your fingertips, the only thing left to do is to get started. The clock is ticking—fire up your editor, and let your next project take shape. Happy coding!
For more inspiration, tutorials, and practical resources, don’t forget to visit Python Developer Resources - Made by 0x3d.site. Your next breakthrough might be just a click away.
???? Download Free Giveaway Products
We love sharing valuable resources with the community! Grab these free cheat sheets and level up your skills today. No strings attached — just pure knowledge! ????
- Nmap - Cheat Sheet - For Beginners/Script Kiddies
- Stealth Tracerouting with 0trace – The Ultimate Cheat Sheet!
- File Compression in Terminal with the Ultimate 7‑Zip Cheat Sheet! ????
- Stealth Network Sniffing with This Ultimate 'Above' Tool Cheat Sheet!
- Advanced Forensic Format (AFF) Toolkit's Ultimate Cheat Sheet
- The Ultimate Aircrack‑ng Cheat Sheet: Crack Wi-Fi Like a Pro (100% Free!) ????????
- Hack Any Software with AFL++! ???? The Ultimate Fuzzing Cheat Sheet (FREE Download)
- Hack Like a Pro: The Ultimate Altdns Cheat Sheet for Subdomain Discovery! ????????
- Hackers Don’t Want You to Know This: The Ultimate Amap Cheat Sheet for Network Recon! ????
- The Ultimate OWASP Amass Cheat Sheet – Master Recon in Minutes! ????
???? More Free Giveaway Products Available Here
- We've 15+ Products for FREE, just get it. We'll promise that you'll learn something out of each.
???? Turn AI Designs into $5,000+/Month with Print-on-Demand!
What if you could use AI-generated designs to create best-selling print-on-demand products and build a passive income stream—without any design skills?
Lifetime Access - Instant Download
With the AI & Print-on-Demand Bundle, you’ll get everything you need to start and scale your business:
- ✅ Step-by-step guide – Learn how to use AI tools like Midjourney, Canva, and Kittl to create high-demand products for Etsy, Shopify, Redbubble, and more.
- ✅ Printable checklist – Follow a proven process covering niche selection, product creation, automation, and scaling so you never miss a step.
- ✅ Exclusive ChatGPT prompts – Generate AI-powered designs, product descriptions, ad copy, and marketing content in seconds.
???? No design skills? No problem. AI does the work—you get the profits!
???? Grab the bundle now and start making sales! Click here to get instant access!